When passing variables to functions, you are duplicating whatever you are
passing. If you set a funciton to take the variables width and height as
input, then when you pass the two values to the function, you actually make
two new variables named height and input, that only lives within the
functions scope. Sometimes there isnt need to make new variables simply
because:
A: The original variable value that is passed
needs to be changed, and creating a new one, then copying the result over to
the original variable would only make the code more complex and slow.
B: You have a small amount of memory, and need
to occupy as little memory as possible.
C: You are changing the data stored in a
specific part of the memory.
There is probarbly dusins of other reasons, but these are the main ones. To
help us in these problems, we have pointers. They are known to be complex
and hard to learn for beginners, but quite frankly I dont see why. A pointer
is, just as the name says, something that "points". The thing it points to,
is memory. Either by directly refering to the memory address, or by refering
to another variable name. Pointers have an asterisk before their name. Now
that was the hard way of explaining it. Let me try another approach; A
pointer is a guy called Larry. Larry have been hired by you, the coder, to
help him guide the application as it either look for or transfer numbers.
The first problem has arrived, as you try to do as follows:
int return_higest_value(int us_citizens_age[])
Clearly, if you wanted to get the age of the oldest person in the US, you
wouldnt want to copy the whole list in memory before doing so. Instead,
Larry is set on the assignment to prevent us from copying the whole list,
and instead just point to where the list really is - which is already in
memory.
int return_higest_value(int *us_citizens_age[])
You can se Larry present in the function shown above. That's right, he's a
star! The application will now just look at the list already stored in
memory. Sounds simple, doesnt it? It sure is, but now its time to get a
little more advanced. You see, as is always the case in computer
programming, you can ALWAYS get more in-depth. Unless you speak fluent
binary of course... Lets take a more in-depth look at what actually happens
in memory when you pass a variable to a function.
Stack Area
The Stack Area, mostly reffered to only as "The Stack", is a special place
in the memory. Whenever you pass a variable to a function - without using a
pointer (which you from now on will know is not the same as passing a
variable), you copy the variable in memory. In other words, you duplicate
it. What, I already said that? Yup, but whats new to this story is that it
isnt just copied "somewhere" in memory, but to a specific place. You guessed
it, its copied to the stack. Copying to the stack is known as "passing data
directly (passing by value)". Its a hard sentence, but you should learn it.
The opposite is "passing data passing its address (passing by reference)".
Thats an even harder sentence, but what it really boils down to is "passing
data using a pointer". Before I go a head and show this to you visually, let
me just explain that the "main" memory, where all the usual variables and
such are stored, is called "Data Area". Now, with that in mind, examine the
following:
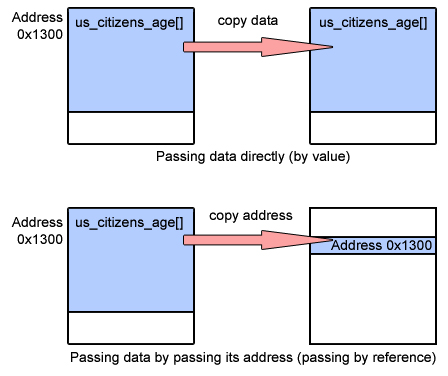
As you can see, the numbers copied over to the stack takes far less space
when passing a pointer. In fact, in most circumstances, it is crucial to do
it this way, or else the application will suffer greatly.
More about pointers in the next part.
|